This Tutorial help to add, edit and delete WordPress post using WP rest API. Already shared What are the WordPress Rest api with Example.I have created word-press post using wordpress api. I am using WordPress REST API plugin for restful interface into WordPress CMS.
I will cover following operation into this WordPress Rest Api tutorial:
- Create New Post using wp-json wordpress plugin
- Update wordpress post using WordPress rest api
- View wordpress post using WordPress rest api
- Delete wordpress post using wp rest api
Table of Contents
Create,Update and Delete WordPress Post using Rest API
I am assuming you have read my previous wordpress tutorial and everything ready to access of WordPress data using API.
Before accessing WP Rest API, We must have username and password to access wordpress api. We also have REST client of any programming languages, You can use Node.js
, django or Guzzle php. I am using Guzzle php client to access WP rest api. You can get more information of Guzzle client from Simple Example Guzzle Rest Client With Rest API tutorial.
Configure Rest Client for WordPress Rest API
I am using Guzzle, So I will pass base64 username:password
string with in rest api request header.The Rest Client header would be like as below,It would be same for all request,
'http://www.yourblogname.com/wp-json/wp/v2/', // You can set any number of default request options. 'timeout' => 2.0, 'headers' => ['Content-Type' => 'application/json', "Accept" => "application/json", 'Authorization' => "Basic " . $base64], //ssl false 'verify' => false ]);
Please change username and password string as per your REST API credentials.The parameters are:
- $base64 : This variable will contains
base64
string of username and password. - base_uri : The WordPress host api url path.
- headers : This will contains request header parameters.
- timeout : Request timeout in sec.
- verify : SSL verification false.
How to create WordPress Post using Rest Service
We have configured REST client and now we will access wordpress rest api using above REST client instance.Lets create a new wordpress post into the database using wordpress rest api. I will create post parameters and passed to guzzle client.We finally send HTTP request to wordpress api.
$params = array( "title" => "Hello Updated World1!", "content_raw" => "Howdy updated content.", "date" => "2017-02-01T14:00:00+10:00" ); $response = $client->post('posts/', [ 'body' => json_encode($params)] ); echo ""; echo $response->getBody();
Created wordpress post data and stored into $params variable.I am using POST HTTP method($client->post()) of Guzzle client and passed data into body of Rest client request.
Once request completed successfully, You will get post data as response which was earlier created by you.The created WordPress post status is DRAFT(default WordPress post status).
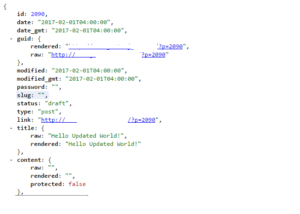
The response code has post id that we will use for further operations into this wordpress tutorial.
Update Post using WordPress Rest API
We will update above wordPress post using rest api. I have created post which are in draft, so let update wordpress post and published using wordpress rest api. I will create post parameters and passed to guzzle client.We finally send request to wordpress Rest API.
$params = array( "status" => "publish", "content" => "Hi, I am created Post by Rest API" ); $response = $client->put('posts/2090', [ 'body' => json_encode($params)] ); echo ""; echo $response->getBody();
as you can see, I have created WordPress post data and stored into $params variable.I am using PUT HTTP method($client->put()
) of Guzzle client and passed data into body of Rest client request and send to server.
Once request has been completed successfully, You will get post data as a response and published into wordpress. You can see newly created post into wordpress dashboard.
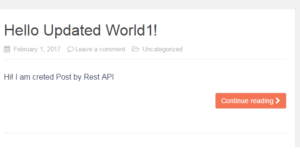
How to Get WordPress Post Information using Rest Service
How to fetch post from WordPress server using rest api? Its very simple just pass post id into rest api with GET type request.You will get all information of single post.
$response = $client->get('posts/2090'); echo " "; echo $response->getBody();
How to Delete WordPress Post using Rest Service
Deleting WordPress post is simple rest request as other json rest api except HTTP method, We will create DELETE type request and send to the server.We will send following request to WordPress API to delete post data from WordPress database.
$response = $client->delete('posts/2090'); echo " "; echo $response->getBody();
I have passed 2090
, which is created post id that we want to delete from WordPress server using rest api. The above code will delete post from WordPress post listing but,post will move into trash, if you want completely delete post from WordPress database, need to use force
argument /wp/v2/posts/2090?force=true
:
$response = $client->delete('posts/2090?force=true'); echo " "; echo $response->getBody();
Conclusion
This Wordpres tutorial help to create wordpress post using wordpress rest api. We have fetched post data, update post data and delete post using rest api. You can implement same for category, media, taxonomies etc.